wasup
Android) 계산기 만들기 본문
반응형
new project name : CalcTest
activity_main.xml
MainActivity.java
만들 화면
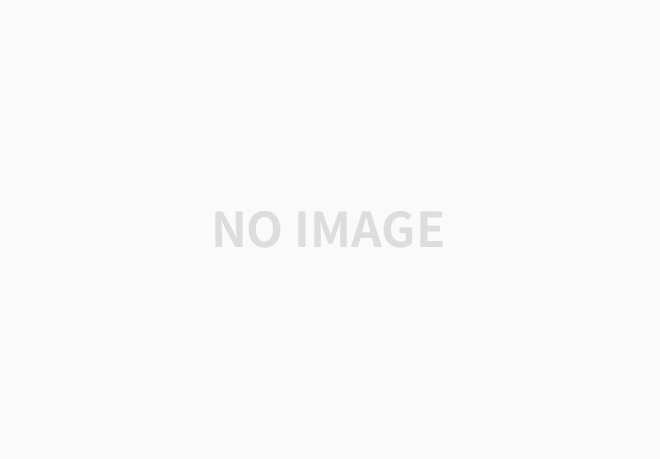
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="#FFFFFF"
android:stretchColumns="0,1,2,3,4"
>
<!--
android:stretchColumns="0,1,2,3,4"
0~4번까지 같은 크기 주기.
-->
<!-- 변수는 소문자로 통일, 자바식 변수명을 따름-->
<TableRow>
<EditText
android:id="@+id/edit1"
android:layout_span="5"
android:hint="숫자1 입력"
/>
</TableRow>
<TableRow>
<EditText
android:id="@+id/edit2"
android:layout_span="5"
android:hint="숫자2 입력"
/>
</TableRow>
<TableRow>
<Button
android:id="@+id/btnNum0"
android:layout_width="50dp"
android:layout_margin="2dp"
android:padding="8dp"
android:textSize="10pt"
android:backgroundTint="#182351"
android:text="0"
/>
<Button
android:id="@+id/btnNum1"
android:layout_width="50dp"
android:layout_margin="2dp"
android:padding="8dp"
android:textSize="10pt"
android:backgroundTint="#182351"
android:text="1"
/>
<Button
android:id="@+id/btnNum2"
android:layout_width="50dp"
android:layout_margin="2dp"
android:padding="8dp"
android:textSize="10pt"
android:backgroundTint="#182351"
android:text="2"
/>
<Button
android:id="@+id/btnNum3"
android:layout_width="50dp"
android:layout_margin="2dp"
android:padding="8dp"
android:textSize="10pt"
android:backgroundTint="#182351"
android:text="3"
/>
<Button
android:id="@+id/btnNum4"
android:layout_width="50dp"
android:layout_margin="2dp"
android:padding="8dp"
android:textSize="10pt"
android:backgroundTint="#182351"
android:text="4"
/>
</TableRow>
<TableRow>
<Button
android:id="@+id/btnNum5"
android:layout_width="50dp"
android:layout_margin="2dp"
android:padding="8dp"
android:textSize="10pt"
android:backgroundTint="#182351"
android:text="5"
/>
<Button
android:id="@+id/btnNum6"
android:layout_width="50dp"
android:layout_margin="2dp"
android:padding="8dp"
android:textSize="10pt"
android:backgroundTint="#182351"
android:text="6"
/>
<Button
android:id="@+id/btnNum7"
android:layout_width="50dp"
android:layout_margin="2dp"
android:padding="8dp"
android:textSize="10pt"
android:backgroundTint="#182351"
android:text="7"
/>
<Button
android:id="@+id/btnNum8"
android:layout_width="50dp"
android:layout_margin="2dp"
android:padding="8dp"
android:textSize="10pt"
android:backgroundTint="#182351"
android:text="8"
/>
<Button
android:id="@+id/btnNum9"
android:layout_width="50dp"
android:layout_margin="2dp"
android:padding="8dp"
android:textSize="10pt"
android:backgroundTint="#182351"
android:text="9"
/>
</TableRow>
<TableRow>
<Button
android:id="@+id/btnAdd"
android:layout_margin="2dp"
android:layout_span="2"
android:padding="5dp"
android:textSize="10pt"
android:backgroundTint="#182351"
android:text="더하기"
/>
<Button
android:id="@+id/btnSub"
android:layout_margin="2dp"
android:layout_span="2"
android:padding="2dp"
android:textSize="10pt"
android:backgroundTint="#182351"
android:text="빼기"
/>
</TableRow>
<TableRow>
<Button
android:id="@+id/btnMul"
android:layout_margin="2dp"
android:layout_span="2"
android:padding="2dp"
android:textSize="10pt"
android:backgroundTint="#182351"
android:text="곱하기"
/>
<Button
android:id="@+id/btnDiv"
android:layout_margin="2dp"
android:layout_span="2"
android:padding="2dp"
android:textSize="10pt"
android:backgroundTint="#182351"
android:text="나누기"
/>
<Button
android:id="@+id/btnCls"
android:layout_margin="2dp"
android:layout_span="1"
android:padding="5dp"
android:textSize="10pt"
android:backgroundTint="#182351"
android:text="cls"
/>
</TableRow>
<TableRow>
<EditText
android:id="@+id/textResult"
android:layout_margin="2dp"
android:layout_span="5"
android:padding="2dp"
android:textSize="10pt"
android:background="#D2DDFF"
android:hint="계산결과"
/>
</TableRow>
</TableLayout>
MainActivity.java
package com.example.calctest;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.MotionEvent;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
//변수
EditText edit1, edit2;
Button btnAdd, btnSub, btnMul, btnDiv, btnCls;
TextView textResult;
String num1, num2; //누를버튼
Integer result; //계산결과
Button[] numButtons = new Button[10]; //배열생성
Integer[] numBtnIDs = {
R.id.btnNum0, R.id.btnNum1, R.id.btnNum2, R.id.btnNum3, R.id.btnNum4,
R.id.btnNum5, R.id.btnNum6, R.id.btnNum7, R.id.btnNum8, R.id.btnNum9
}; //버튼 10개의 아이디
int i;//이벤트 끝난다음 for문에서 사용
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);//activity_main.xml
setTitle("계산기");
//id값 가져오기
edit1=(EditText) findViewById(R.id.edit1);//edit1 id값 가져오기
edit2=(EditText) findViewById(R.id.edit2);//edit2 id값 가져오기
btnAdd=(Button) findViewById(R.id.btnAdd);//btnAdd id값 가져오기
btnSub=(Button) findViewById(R.id.btnSub);//btnSub id값 가져오기
btnMul=(Button) findViewById(R.id.btnMul);//btnMul id값 가져오기
btnDiv=(Button) findViewById(R.id.btnDiv);//btnDiv id값 가져오기
btnCls=(Button) findViewById(R.id.btnCls);//btnCls id값 가져오기
textResult=(TextView) findViewById(R.id.textResult);//textResult id값 가져오기
//연산버튼 터치 이벤트-더하기
btnAdd.setOnTouchListener(new View.OnTouchListener() {
@Override
public boolean onTouch(View view, MotionEvent motionEvent) {
num1 = edit1.getText().toString();
num2 = edit2.getText().toString();
if(num1.length()<1 || num2.length()<1){//길이가 1보다 작으면(없으면)
Toast.makeText(getApplicationContext(), "먼저 숫자를 선택(입력)하세요.", Toast.LENGTH_SHORT).show();
return false;
}//if-end
result=Integer.parseInt(num1)+Integer.parseInt(num2);//더하기 연산
textResult.setText("연산결과"+result.toString());//연산결과
return false;
}
});//btnAdd-event-end
//연산버튼 터치 이벤트-빼기
btnSub.setOnTouchListener(new View.OnTouchListener() {
@Override
public boolean onTouch(View view, MotionEvent motionEvent) {
num1 = edit1.getText().toString();
num2 = edit2.getText().toString();
if(num1.length()<1 || num2.length()<1){//길이가 1보다 작으면(없으면)
Toast.makeText(getApplicationContext(), "먼저 숫자를 선택(입력)하세요.", Toast.LENGTH_SHORT).show();
return false;
}//if-end
result=Integer.parseInt(num1)-Integer.parseInt(num2);//빼기 연산
textResult.setText("연산결과"+result.toString());//연산결과
return false;
}
});//btnSub-event-end
//연산버튼 터치 이벤트-곱하기
btnMul.setOnTouchListener(new View.OnTouchListener() {
@Override
public boolean onTouch(View view, MotionEvent motionEvent) {
num1 = edit1.getText().toString();
num2 = edit2.getText().toString();
if(num1.length()<1 || num2.length()<1){//길이가 1보다 작으면(없으면)
Toast.makeText(getApplicationContext(), "먼저 숫자를 선택(입력)하세요.", Toast.LENGTH_SHORT).show();
return false;
}//if-end
result=Integer.parseInt(num1)*Integer.parseInt(num2);//빼기 연산
textResult.setText("연산결과"+result.toString());//연산결과
return false;
}
});//btnMul-event-end
//연산버튼 터치 이벤트-나누기
btnDiv.setOnTouchListener(new View.OnTouchListener() {
@Override
public boolean onTouch(View view, MotionEvent motionEvent) {
num1 = edit1.getText().toString();
num2 = edit2.getText().toString();
if(num1.length()<1 || num2.length()<1){//길이가 1보다 작으면(없으면)
Toast.makeText(getApplicationContext(), "먼저 숫자를 선택(입력)하세요.", Toast.LENGTH_SHORT).show();
return false;
}//if-end
result=Integer.parseInt(num1)/Integer.parseInt(num2);//빼기 연산
textResult.setText("연산결과"+result.toString());//연산결과
return false;
}
});//btnDiv-event-end
//연산버튼 터치 이벤트-Cls
btnCls.setOnTouchListener(new View.OnTouchListener() {
@Override
public boolean onTouch(View view, MotionEvent motionEvent) {
edit1.setText("");//숫자선택1
edit2.setText("");//숫자선택2
textResult.setText("");//연산결과
return false;
}
});//btnCls-event-end
//숫자 버튼을 클릭하여 데이터 입력하기
for(i=0; i<numBtnIDs.length; i++){//버튼 배열 길이만큼 반복
numButtons[i]=(Button) findViewById(numBtnIDs[i]); //버튼에 아이디값 넣기
}//for-end
//버튼 선택시 변수
for(i=0; i<numBtnIDs.length; i++){//버튼 배열 길이만큼
final int index; //변수
index=i;
numButtons[index].setOnClickListener(new View.OnClickListener() {//버튼에 버튼 배열 길이만큼 event반복
@Override
public void onClick(View view) {
if(edit1.isFocused()==true){//edit1텍스트에디터에 포커싱이 잡히면
num1=edit1.getText().toString()+numButtons[index].getText().toString();//num1에 edit1텍스트에디터를 String으로 변경+버튼의 텍스트를 String으로 변경
edit1.setText(num1);//
}else if(edit2.isFocused()==true){//edit2텍스트에디터에 포커싱이 잡히면
num2=edit2.getText().toString()+numButtons[index].getText().toString();//num2에 edit2텍스트에디터를 String으로 변경+버튼의 텍스트를 String으로 변경
edit2.setText(num2);//
}else{//edit1, edit2에 포커싱 없으면
Toast.makeText(getApplicationContext(), "먼저 텍스트영역을 선택하세요", Toast.LENGTH_SHORT).show();
}//if-else-if-end
}//onClick-end
});//numButtons[index]-end
}//for-end
}//onCreate-end
}//class-end
.isFocused()를 .isFocusable()로 잘못사용해서 edit1에만 숫자가 입력되는 슬픈일이 있었음.
.isFocusable() 메소드를 통해 포커스를 가질 수 있는지의 여부를 확인
// 사용가능 여부
view.setEnabled(true);
view.isEnabled();
// 선택여부
view.setSelected(true);
view.isSelected();
// 포커스 여부
view.setFocusable(true);
view.isFocusable();
// 눌림 여부
view.setPressed(true);
view.isPressed();
참고링크
반응형
'IT > Android' 카테고리의 다른 글
Android) 버튼클릭시 각각 다른 화면으로 이동! (0) | 2021.08.20 |
---|---|
Android) Test (0) | 2021.08.14 |
Android) WebView (0) | 2021.08.07 |
Android) ActionBar.TabListener (0) | 2021.08.07 |
Android) TabHost (0) | 2021.08.07 |